SSH Tunnels / ProxyJump
SSH Tunnels Overview
I completely missed that ProxyJump exists!
SSH tunnels forward traffic through an encrypted SSH channel. There are three main types:
- Local Forwarding: Redirects a local port to a remote service.
- Remote Forwarding: Exposes a remote port locally.
- Dynamic Forwarding: Acts as a SOCKS proxy for dynamic routing.
Traditional Tunneling: ProxyCommand
Before ProxyJump, multi-hop tunneling used the ProxyCommand
directive:
Host target
HostName target.example.com
User user
ProxyCommand ssh -W %h:%p jump.example.com
While functional, this approach can be verbose and less intuitive.
ProxyJump: Simplified Multi-Hop Tunneling
Introduced in OpenSSH 7.3, ProxyJump streamlines chaining:
Host target
HostName target.example.com
User user
ProxyJump jump.example.com
ProxyJump automatically sets up the intermediary connection, reducing complexity.
Chaining Multiple Jump Hosts
You can specify multiple hops with a comma-separated list:
Host target
HostName target.example.com
User user
ProxyJump jump1.example.com,jump2.example.com
This config connects first to jump1
, then to jump2
, before reaching the target.
SSHFS and ProxyJump
SSHFS leverages the SSH configuration, so you can mount remote filesystems through jump hosts without extra scripting. Simply reference the host defined in your SSH config.
Key Benefits of ProxyJump
- Simplicity: Cleaner, more readable configuration.
- Flexibility: Easily chain multiple hosts.
- Maintenance: Reduces the need for complex command-line options.
This modern approach makes setting up secure, multi-hop SSH connections straightforward and robust.
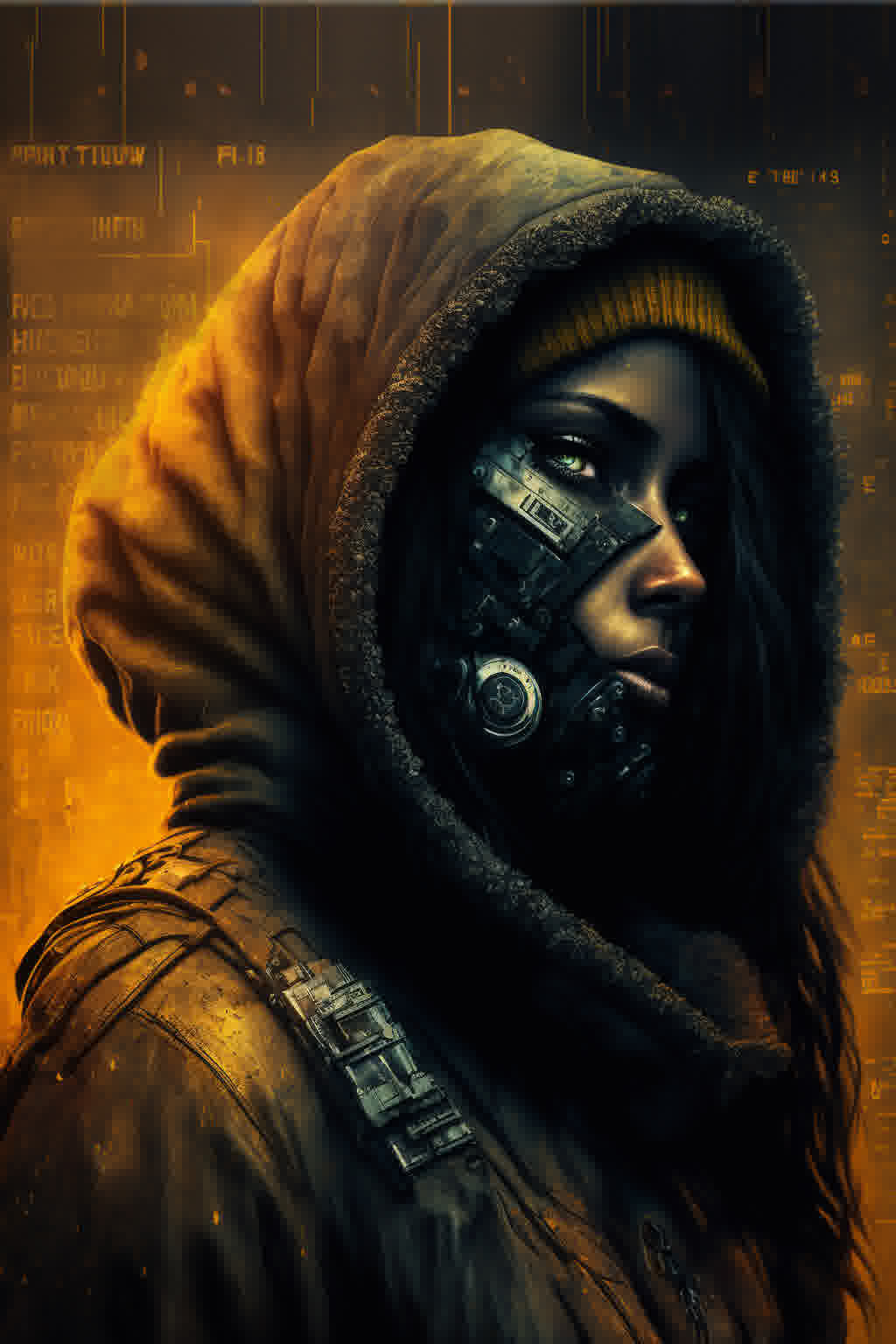